Dot Operator
The useful methods on arrays—iterating over elements, searching, sorting, filling, filtering, and so on—are all provided as methods on slices, not arrays. Rust implicitly converts an array to a slice when searching for methods.
Consider the following code:
|
|
In the last two lines, Rust automatically converts the &Vec<f64>
reference and the &[f64; 4]
reference to slice references that point directly to the data. By the end, memory looks like this:
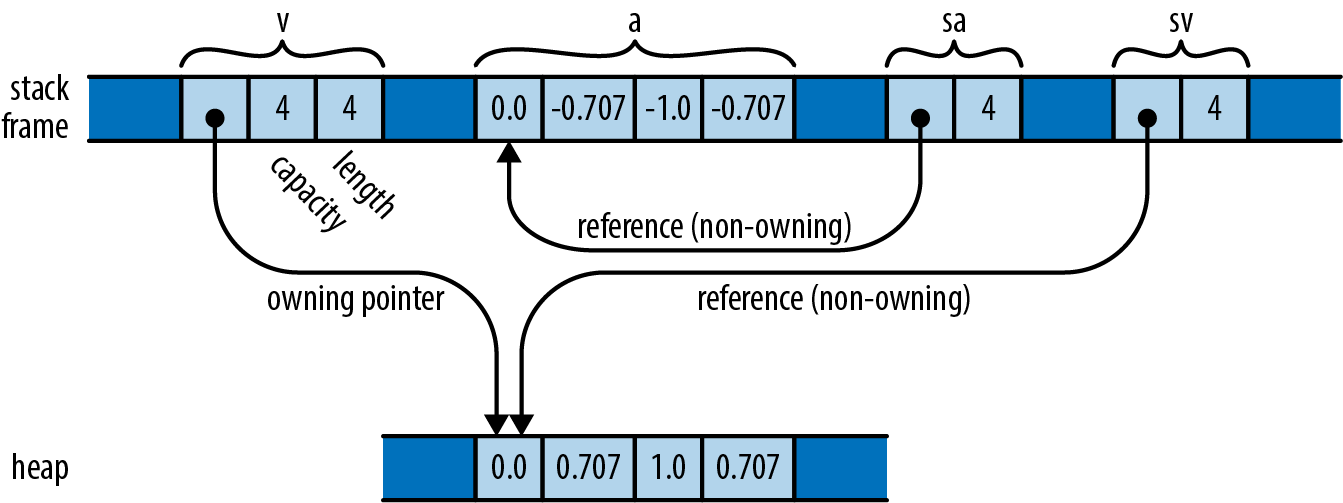
The reverse
method is actually defined on slices, but the call implicitly borrows a &mut [&str]
slice from the vector and invokes reverse
on that:
|
|
Rust implicitly produces a &mut [i32]
slice referring to the entire array and passes that to sort
to operate on:
|
|
References
In Rust, references are created explicitly with the &
operator, and dereferenced explicitly with the *
operator. The .
operator is an exception. It borrows and dereferences implicitly.
|
|
Deref Coercions
A conversion usually requires a cast. A few conversions involving reference types are so straightforward that the language performs them even without a cast:
- Values of type
&String
auto-convert to type&str
without a cast. - Values of type
&Vec<i32>
auto-convert to&[i32]
. - Values of type
&Box<Chessboard>
auto-convert to&Chessboard
. - Values of a
mut
reference auto-convert to a non-mut
reference without a cast.
These are called deref coercions, because they apply to types that implement the Deref
built-in trait. The purpose of Deref
coercion is to make smart pointer types, like Box
, behave as much like the underlying value as possible. Using a Box<Chessboard>
is mostly just like using a plain Chessboard
, thanks to Deref
.